State Machine Modeling
Contents
State Machine Modeling#
State Machine Modeling Concepts#
The ZDL language can be used to specify a state machine, or automaton, for a protocol. A state machine is based on two components: States and Transitions. A state represents the status of a service, and expects conditions to trigger the execution of a transition. A transition is a list of actions that will be executed when a condition is met at a specific state (such as the receipt of a network message). The list of actions may contain sending a network message, changing the value of session or global variables, moving to another state in the automaton, etc.
The language defines three kinds of transition in an automaton:
Standard transitions: this represents a transition between two states (an initial state and an end state) in an automaton. The initial state and the end state can be the same.
Opening channel transitions: this represents a transition which, when executed, requests to open the current underlying communication channel.
Closing channel transitions: this represents a transition which, when executed, requests to close the current underlying communication channel.
In the Netzob library, a state machine relies on symbols to trigger transitions between states. In order to represent the state machine structure, the library relies on a mathematical model based on a Mealy machine (cf. https://en.wikipedia.org/wiki/Mealy_machine). The library leverages this model by associating, for each transition, an input symbol and a list of output symbols, as shown on the figure below.
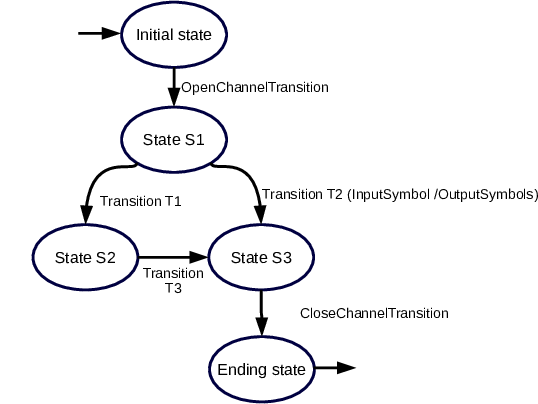
Example of State Machine modeling with states, transitions, input and output symbols.#
Depending on the peer point of view, either an initiator (e.g. a client that starts a communication with a remote service) or a non initiator (e.g. a service that waits for input messages), the interpretation of the state machine is different. This intepretation is done with a state machine visitor that is called an Actor
in the API.
From an initiator point of view, when the actor is at a specific state in the automaton, a random transition is taken amongst the available transitions. In the above example, two transitions, T1
and T2
, are available at the state S1
. Then, the input symbol of the picked transition is specialized into a message and this message is emitted to the target. If the target replies, the actor abstracts the received message into a symbol, and checks if this symbol corresponds to one of the expected output symbols. If it matches, the transition succeeds and thus leads to the end state of the transition. In the above example, the transition T2
would lead to the state S3
. If no response comes from the target, or if a wrong message is received, we leave the automaton.
From a non initiator point of view, when at a specific state in the automaton, the actor waits for a network message. When one network message is received, it is abstracted into a symbol. Then, we retrieve the transition that has this symbol as input symbol. When a transition is retrieved, we randomly pick one symbol amongst the output symbols, and send this symbol to the remote peer. Finally, the transition leads to the end state of the transition.
When the actor has to select a transition, or when the actor has to identify the current transition according to the received message, it is possible to influence this choice through the help of callback functions.
Likewise, when the actor sends the input symbol, or when the actor sends an output symbol, it is possible to influence the selection of the symbol, through the help of callback functions or selection probability weight.
In order to model hybrid state machines where a peer is able to send or receive symbols depending on the context, it is possible to change the initiator behavior at specific transitions. This is done through the inverseInitiator
attribute on Transition
objects. When setting this attribute to True
on a transition, an actor will inverse the way symbols are exchanged (e.g. an initiator actor will first wait for an input symbol and then send one of the output symbols).
A Memory (see Memory
) is used to keep track of a context for a specific communication. This memory can leverage variable from the protocol or even the environment. The memory is initialized at the beginning of the communication, and its internal state evolves throughout the exchanged messages.
Modeling States#
In the API, automaton states are modeled through the State class.
- class State(name=None)[source]#
This class represents a state in an automaton.
The State constructor expects some parameters:
- Parameters
name (
str
, optional) – The name of the state. If None, it is set to ‘State’.
The State class provides the following public variables:
- Variables
name (
str
) – The name of the state. The default value is ‘State’.transitions (List[Transition]) – The list of outgoing transitions
The following example shows the definition of an
s0
state and ans1
state:>>> from netzob.all import * >>> s0 = State() >>> s0.name 'State' >>> s1 = State(name="S1") >>> s1.name 'S1'
Modeling Transitions#
The available transitions are detailed in this chapter.
- class Transition(startState, endState, inputSymbol=None, outputSymbols=None, name=None)[source]#
This class represents a transition between two states (an initial state and an end state) in an automaton. The initial state and the end state can be the same.
A transition is either in an initiator or non initiator context. In an initiator context, the input symbol of the transition is emitted and one of the output symbols of the transition is expected. In non initiator context, the input symbol of the transition is expected and one of the output symbols of the transition is emitted.
The context of the transition (either initiator or non initiator) is defined from the actor type that visits the automaton and from the value of the
inverseInitiator
attribute of the transition (which is by default set toFalse
). The context is defined as follows:if the actor has its
initiator
attribute set toTrue
, and if theinverseInitiator
attribute is set toTrue
, the transition context isinitiator
;if the actor has its
initiator
attribute set toTrue
, and if theinverseInitiator
attribute is set toFalse
, the transition context isnon initiator
;if the actor has its
initiator
attribute set toFalse
, and if theinverseInitiator
attribute is set toTrue
, the transition context isinitiator
;if the actor has its
initiator
attribute set toFalse
, and if theinverseInitiator
attribute is set toFalse
, the transition context isnon initiator
.
When an actor encounters a state where multiple transitions are defined, a random choice is made amongst all these transitions by considering their probabilities. Two scenarios are possible:
if the picked transition is in an
initiator
context, this transition is executed;otherwise, the executed transition depends on the received symbol.
It is possible to define probability on transition selection during state processing, through the
inputSymbolProbability
attribute. This functionality makes it possible to implement a variety of state machines.Note
In a state, if several transitions are available, where some of them expect to receive the input symbol (non initiator context) and the others expect to send the input symbol (initiator context), it is recommended in the last case to not set any output symbols to be received. In such a situation, it is better to handle the receiving of the potential peer symbols in a second state.
Two transitions in the same context, initiator or non initiator, that start at the same state cannot have the same input symbol, as this symbol is used to determine the corresponding transition when receiving a new message. The only exception is when the transitions that have the same input symbol leverage the
inputSymbolPreset
attribute. In such case, theinputSymbolPreset
attribute makes it possible to determine the corresponding transition based on field content from the same input symbol.The Transition constructor expects some parameters:
- Parameters
startState (
State
, required) – This parameter is the initial state of the transition.endState (
State
, required) – This parameter is the end state of the transition.inputSymbol (
Symbol
, optional) – The input symbol which triggers the execution of the transition. The default value isNone
, which means that no symbol is expected in a non initiator context, and no symbol is sent in an initiator context. Internally, None symbol will be replaced by anEmptySymbol
.outputSymbols (a
list
ofSymbol
, optional) – A list of expected output symbols when the current transition is executed. The default value isNone
, which means that no symbol will be sent in a non initiator context, and no symbol is expected in an initiator context. Internally,None
symbol will be replaced by anEmptySymbol
.name (
str
, optional) – The name of the transition. The default value is None.
The Transition class provides the following public variables:
- Variables
startState (
State
) – The initial state of the transition.endState (
State
) – The end state of the transition.inputSymbolProbability (
float
) – This value holds the probability of the current transition of being chosen when processing the state where it is attached. The value between0.0
and100.0
corresponds to the weight of the transition in terms of selection probability. The default value is set to 10.0.inputSymbol (
Symbol
) – The input symbol is the symbol which triggers the execution of the transition.outputSymbols (
list
ofSymbol
) – Output symbols that can be generated or expected when the current transition is executed.inputSymbolPreset (
Preset
) – A preset configuration used during specialization and abstraction of symbols emitted and received. During specialization, values in this configuration will override any field definition, constraints or relationship dependencies. During abstraction, this structure is used to validate the data of the received symbol. If the data does not match, we leave the automaton or execute the function set by theset_cbk_read_unexepected_symbol()
method. SeePreset
for a complete explanation of Preset usage.outputSymbolsPreset (
dict
ofPreset
) – Adict
of preset configurations used during specialization and abstraction of symbols emitted and received. During specialization, values in this configuration will override any field definition, constraints or relationship dependencies. During abstraction, this structure is used to validate the data of the received symbol. If the data does not match, we leave the automaton or execute the function set by theset_cbk_read_unexepected_symbol()
method. SeePreset
for a complete explanation of Preset usage.name (
str
) – The name of the transition.inputSymbolReactionTime (
float
) – The timeout value in seconds to wait for the input value (only used in a non initiator context).outputSymbolsReactionTime (
dict
{Symbol
,float
}) – Adict
containing, for each output symbol, the timeout value in seconds to wait for the output value (only used in an initiator context).outputSymbolsProbabilities (
dict
{Symbol
,float
}) – A structure that holds the selection probability of each symbol as an output symbol. The value between0.0
and100.0
corresponds to the weight of the symbol in terms of selection probability. The default value of each symbol as an output symbol is set to 10.0.inverseInitiator (
bool
) – Indicates to inverse the behavior of the actorinitiator
attribute.description (
str
) – The description of the transition. If not explicitly set, it is generated from the input and output symbol strings.
The following example shows the definition of a transition t between two states, s0 and s1:
>>> from netzob.all import * >>> s0 = State() >>> s1 = State() >>> t = Transition(s0, s1, name="transition")
The following code shows access to attributes of a Transition:
>>> print(t.name) transition >>> s0 == t.startState True >>> s1 == t.endState True
The following example shows the definition of a named Transition that accepts a specific input symbol and produces an output symbol from a list that contains one symbol element:
>>> from netzob.all import * >>> t = Transition(State(), State(), name="testTransition") >>> t.inputSymbol = Symbol() >>> t.outputSymbols = [Symbol()]
The following example shows the definition of a state with two transitions that have a different probability. Here, the transition T2 is twice as likely to be chosen as T1.
>>> from netzob.all import * >>> s0 = State() >>> s1 = State() >>> s2 = State() >>> t1 = Transition(s0, s1, name="T1") >>> t1.inputSymbolProbability = 20.0 >>> t2 = Transition(s0, s2, name="T2") >>> t2.inputSymbolProbability = 40.0
- class OpenChannelTransition(startState, endState, name=None)[source]#
This class represents a transition which, when executed, requests to open the underlying communication channel that the actor uses to exchange messages with the peer (i.e. a call to the
open()
method of the channel is made). The starting state of this transition corresponds to the initial state of the automaton.The OpenChannelTransition expects some parameters:
- Parameters
startState (
State
, required) – This parameter is the initial state of the transition. This also corresponds to the initial state of the automaton.endState (
State
, required) – This parameter is the end state of the transition.name (
str
, optional) – The name of the transition. The default value is OpenChannelTransition.
The OpenChannelTransition class provides the following public variables:
- Variables
The following example shows the creation of an OpenChannelTransition transition:
>>> from netzob.all import * >>> s0 = State() >>> s1 = State() >>> t = OpenChannelTransition(s0, s1, name="transition") >>> print(t.name) transition >>> s0 == t.startState True >>> s1 == t.endState True
- class CloseChannelTransition(startState, endState, name=None)[source]#
This class represents a transition which, when executed, requests to close the underlying communication channel that the actor uses to exchange messages with the peer (i.e. a call to the
close()
method of the channel is made). The ending state of this transition corresponds to a terminal state of the automaton. From an automaton point of view, we can have multiple CloseChannelTransition, and therefore we can have multiple terminal states in the automaton.The CloseChannelTransition expects some parameters:
- Parameters
startState (
State
, required) – This parameter is the initial state of the transition.endState (
State
, required) – This parameter is the ending state of the transition. This also corresponds to an ending state of the automaton.name (
str
, optional) – The name of the transition. The default value is CloseChannelTransition
The CloseChannelTransition class provides the following public variables:
- Variables
The following example shows the creation of a CloseChannelTransition transition:
>>> from netzob.all import * >>> s0 = State() >>> s1 = State() >>> t = CloseChannelTransition(s0, s1, name="transition") >>> print(t.name) transition >>> print(s0 == t.startState) True >>> print(s1 == t.endState) True
Taking Control over Emitted Symbol and Selected Transition#
A state may have different available transitions to other states. It
is possible to filter those available transitions in order to limit
them or to force a specific transition to be taken. The filtering
capability works by adding callbacks through the
add_cbk_filter_transitions()
method on a
State
instance.
- State.add_cbk_filter_transitions(cbk_method)#
Add a function called during state execution to filter the available transitions. Callbacks defined through this method are executed before the random choice made by the actor, amongst the available transitions, when it visits the current state of the automaton.
- Parameters
cbk_method (
Callable
, required) – the callback function- Raise
TypeError
ifcbk_method
is not a callable function
The callback function that can be used in the
cbk_method
parameter has the following prototype:- cbk_method(available_transitions, current_state, last_sent_symbol, last_sent_message, last_sent_structure, last_received_symbol, last_received_message, last_received_structure, memory)
- Parameters
available_transitions (List[Transition]) – Corresponds to the list of available transitions starting from the current state.
current_state (
State
) – Current state in the automaton.last_sent_symbol (
Symbol
) – Last sent symbol by the actor on the communication channel, and thus making it possible to create relationships with the previously sent symbol.last_sent_message (
bitarray
) – Corresponds to the last sent message by the actor on the communication channel, and thus making it possible to create relationships with the previously sent message.last_sent_structure (
OrderedDict
where keys areField
and values arebytes
) – Corresponds to the last sent message structure by the actor on the communication channel, and thus making it possible to create relationships with the previously sent message structure.last_received_symbol (
Symbol
) – Corresponds to the last received symbol by the actor on the communication channel, and thus making it possible to create relationships with the previously received symbol.last_received_message (
bitarray
) – Corresponds to the last received message by the actor on the communication channel, and thus making it possible to create relationships with the previously received message.last_received_structure (
OrderedDict
where keys areField
and values arebytes
) – Corresponds to the last received message structure by the actor on the communication channel, and thus making it possible to create relationships with the previously received message structure.memory (
Memory
) – Corresponds to the current memory context.
- Returns
The callback function should return a list of transition objects.
- Return type
list
ofTransition
When a transition is selected, it is possible to modify it by adding
callbacks through the add_cbk_modify_transition()
method on a
State
instance.
- State.add_cbk_modify_transition(cbk_method)#
Add a function called during state execution to help modify the current transition. The current transition may therefore be replaced by a new transition. The transition returned by the callback should have its
inverseInitiator
attribute set to the same value as for the current transition.Depending on the context of the current transition, the transition modification has a different impact:
If the current transition has its context defined to be
initiator
, the input symbol of the new transition is emitted and one of the output symbols of the new transition is expected. The exiting state corresponds to the ending state of the new transition.If the current transition has its context defined to be
non initiator
, as the input symbol of the current transition has already been received when the callback is called, it only remains to emit one of the output symbols of the new transition. The exiting state corresponds to the ending state of the new transition.
- Parameters
cbk_method (
Callable
, required) – the callback function- Raise
TypeError
ifcbk_method
is not a callable function
The callback function that can be used in the
cbk_method
parameter has the following prototype:- cbk_method(available_transitions, current_transition, current_state, last_sent_symbol, last_sent_message, last_sent_structure, last_received_symbol, last_received_message, last_received_structure, memory)
- Parameters
available_transitions (List[Transition]) – Corresponds to the list of available transitions starting from the current state.
current_transition (
Transition
) – Current transition in the automaton.current_state (
State
) – Current state in the automaton.last_sent_symbol (
Symbol
) – Last sent symbol by the actor on the communication channel, and thus making it possible to create relationships with the previously sent symbol.last_sent_message (
bitarray
) – Corresponds to the last sent message by the actor on the communication channel, and thus making it possible to create relationships with the previously sent message.last_sent_structure (
OrderedDict
where keys areField
and values arebytes
) – Corresponds to the last sent message structure by the actor on the communication channel, and thus making it possible to create relationships with the previously sent message structure.last_received_symbol (
Symbol
) – Corresponds to the last received symbol by the actor on the communication channel, and thus making it possible to create relationships with the previously received symbol.last_received_message (
bitarray
) – Corresponds to the last received message by the actor on the communication channel, and thus making it possible to create relationships with the previously received message.last_received_structure (
OrderedDict
where keys areField
and values arebytes
) – Corresponds to the last received message structure by the actor on the communication channel, and thus making it possible to create relationships with the previously received message structure.memory (
Memory
) – Corresponds to the current memory context.
- Returns
The callback function should return a transition object. The returned transition should have its
inverseInitiator
attribute set to the same value as for the current transition.- Return type
Besides, during execution of a transition, it is possible to change
the symbol that will be sent to the remote peer, by adding callbacks
through the add_cbk_modify_symbol()
method on a
Transition
instance.
- Transition.add_cbk_modify_symbol(cbk_method)#
Function called during transition execution, to help choose/modify the output symbol to send (in a non initiator context) or the input symbol to send (in an initiator context).
- Parameters
cbk_method (Callable, required) – the callback function
- Raise
TypeError
ifcbk_method
is not a callable function
The callback function that can be used in the
cbk_method
parameter has the following prototype:- cbk_method(available_symbols, current_symbol, current_symbol_preset, current_state, last_sent_symbol, last_sent_message, last_sent_structure, last_received_symbol, last_received_message, last_received_structure, memory)
- Parameters
available_symbols (List[Symbol]) – Corresponds to the list of possible symbols to send.
current_symbol (
Symbol
) – Currently selected symbol that will be sent, either the initial symbol or the symbol returned by the previous callback.current_symbol_preset (
Preset
) – Preset configuration associated to selected symbol.current_state (
State
) – Current state in the automaton.last_sent_symbol (
Symbol
) – This parameter is the last sent symbol by the actor on the communication channel, and thus making it possible to create relationships with the previously sent symbol.last_sent_message (
bitarray
) – This parameter is the last sent message by the actor on the communication channel, and thus making it possible to create relationships with the previously sent message.last_sent_structure (
OrderedDict
where keys areField
and values arebytes
) – This parameter is the last sent message structure by the actor on the communication channel, and thus making it possible to create relationships with the previously sent message structure.last_received_symbol (
Symbol
) – This parameter is the last received symbol by the actor on the communication channel, and thus making it possible to create relationships with the previously received symbol.last_received_message (
bitarray
) – This parameter is the last received message (bitarray
) by the actor on the communication channel, and this makes it possible to create relationships with received message.last_received_structure (
OrderedDict
where keys areField
and values arebytes
) – This parameter is the last received message structure by the actor on the communication channel, and thus making it possible to create relationships with the previously received message structure.memory (
Memory
) – This parameter corresponds to the current memory context.
- Returns
The callback function should return a tuple. The first tuple element is the symbol (
Symbol
) that will be sent. This could be the same as thecurrent_symbol
or another one. The second tuple element is a preset (Preset
) configuration used to parameterize fields during symbol specialization. This configuration will override any field definition, constraints or relationship dependencies (seespecialize()
, for more information).- Return type
Executing Actions during Transitions#
It is possible to execute specific actions during transitions, after sending or receiving a symbol, by adding
callbacks through the add_cbk_action()
method on a
Transition
instance. The typical usage of this callback is that it is possible to manipulate the memory context of the automaton after sending or receiving a symbol.
When specifying such callback on a transition, this callback is then called twice for a transition: in an initiator context, the callback is first called after sending the input symbol, and then called after receiving one of the output symbols; while in a non initiator context, the callback is called after receiving the input symbol, and then called after sending one of the output symbols.
- Transition.add_cbk_action(cbk_method)[source]#
Function called after sending or receiving a symbol in the transition. This function should be used to modify the memory context.
- Parameters
cbk_method (
Callable
, required) – the callback function- Raise
TypeError
ifcbk_method
is not a callable function
The callback function that can be used in the
cbk_method
parameter has the following prototype:- cbk_method(symbol, data, data_structure, operation, current_state, memory)
- Parameters
symbol (
Symbol
) – Corresponds to the last sent or received symbol.data (
bytes
) – Corresponds to the last sent or received data.data_structure (
OrderedDict
) – Corresponds to the last sent or received data structure.operation (
Operation
) – Tells the way the symbol is manipulated: eitherOperation.ABSTRACT
for symbols that are abstracted orOperation.SPECIALIZE
for symbols that are specialized.current_state (
State
) – Current state in the automaton.memory (
Memory
) – Corresponds to the current memory context.
The callback method is not expected to return something.
Summary of States and Transitions Processing#
The following figure gives a summary of the sequence of operations during states and transitions processing.
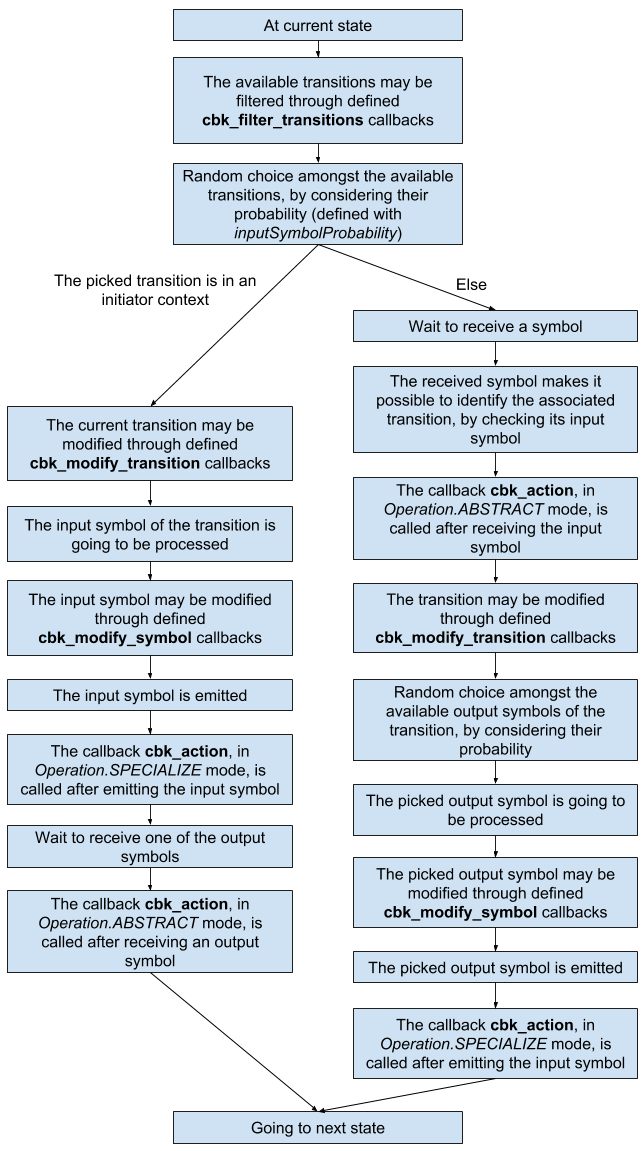
Sequence of operations during states and transitions processing#
Modeling Automata#
In the API, an automaton is made of a list of permitted symbols and an initial state. An automaton is modeled using the Automata class.
- class Automata(initialState, symbols)[source]#
Class which describes an automaton (i.e. state machine) of a protocol. The underlying structure of the automaton is a Mealy machine (cf. https://en.wikipedia.org/wiki/Mealy_machine).
The Automata constructor expects some parameters:
- Parameters
The Automata class provides the following public variables:
- Variables
The following example shows the definition of an automaton with three states s0, s1, s2, an opening transition between states s0 and s1, a standard transition within the same state s1 which accepts the input symbol inputSymbol and generate the output symbol outputSymbol, and a closing transition between states s1 and s2.
>>> # Creation of some states and transitions >>> from netzob.all import * >>> s0 = State(name="S0") >>> s1 = State(name="S1") >>> s2 = State(name="S2") >>> openTransition = OpenChannelTransition(startState=s0, endState=s1, name="Open") >>> inputSymbol = Symbol() >>> outputSymbol = Symbol() >>> mainTransition = Transition(startState=s1, endState=s1, inputSymbol=inputSymbol, outputSymbols=[outputSymbol], name="hello") >>> closeTransition = CloseChannelTransition(startState=s1, endState=s2, name="Close") >>> # Creation of the automata >>> automata = Automata(s0, [inputSymbol, outputSymbol])
- copy()[source]#
Copy the current automaton.
This method copies the states and transitions of the automaton, but keeps references to the original callbacks and symbols.
- Returns
A new object of the same type.
- Return type
>>> # Creation of some states and transitions >>> from netzob.all import * >>> s0 = State(name="S0") >>> s1 = State(name="S1") >>> s2 = State(name="S2") >>> openTransition = OpenChannelTransition(startState=s0, endState=s1, name="open transition") >>> inputSymbol = Symbol() >>> outputSymbol = Symbol() >>> mainTransition = Transition(startState=s1, endState=s1, inputSymbol=inputSymbol, outputSymbols=[outputSymbol], name="main transition") >>> closeTransition = CloseChannelTransition(startState=s1, endState=s2, name="close transition") >>> # Creation of the automata >>> automata = Automata(s0, [inputSymbol, outputSymbol]) >>> automata_bis = automata.copy()
- generateASCII()[source]#
Render the ASCII representation of the automaton.
- Returns
A string containing an ASCII representation of the automaton.
- Return type
str
- generateDotCode()[source]#
Generates the dot code representing the automaton.
- Returns
A string containing a dot code representation of the automaton.
- Return type
str
>>> # Create some states and transitions >>> from netzob.all import * >>> s0 = State(name="S0") >>> s1 = State(name="S1") >>> s2 = State(name="S2") >>> openTransition = OpenChannelTransition(startState=s0, endState=s1, name="Open") >>> inputSymbol = Symbol() >>> outputSymbol = Symbol() >>> mainTransition = Transition(startState=s1, endState=s1, inputSymbol=inputSymbol, outputSymbols=[outputSymbol], name="hello") >>> closeTransition = CloseChannelTransition(startState=s1, endState=s2, name="Close")
>>> # Create the automaton >>> automata = Automata(s0, [inputSymbol, outputSymbol]) >>> print(automata.generateDotCode()) digraph G { "S0" [shape=doubleoctagon, label="S0", style=filled, fillcolor=white, URL="..."]; "S1" [shape=ellipse, label="S1", style=filled, fillcolor=white, URL="..."]; "S2" [shape=ellipse, label="S2", style=filled, fillcolor=white, URL="..."]; "S0" -> "S1" [fontsize=5, label="OpenChannelTransition", URL="..."]; "S1" -> "S1" [fontsize=5, label="hello (Symbol;{Symbol})", URL="..."]; "S1" -> "S2" [fontsize=5, label="CloseChannelTransition", URL="..."]; }
- Returns
a string containing the dot code of the automata.
- Return type
a
list
- getState(name)[source]#
Returns the State object of the given name.
- Parameters
name (
str
, required) – The name of the State object- Returns
The State object with stateName as name.
- Return type
- Raise
KeyError
if the name is not found.
- getStates(main_states=False)[source]#
Visits the automata to discover all the available states.
- Parameters
main_states (
bool
, optional) – Specify that all states except the initial state and the closing states are returned. Default value isFalse
, meaning that all states are returned.- Returns
A list containing all the automaton states.
- Return type
a
list
ofState
>>> from netzob.all import * >>> # Create some states and transitions >>> s0 = State(name="State 0") >>> s1 = State(name="State 1") >>> s2 = State(name="State 2") >>> openTransition = OpenChannelTransition(startState=s0, endState=s1, name="Open") >>> inputSymbol = Symbol() >>> outputSymbol = Symbol() >>> mainTransition = Transition(startState=s1, endState=s1, inputSymbol=inputSymbol, outputSymbols=[outputSymbol], name="hello") >>> closeTransition = CloseChannelTransition(startState=s1, endState=s2, name="Close") >>> # Create the automata >>> automata = Automata(s0, [inputSymbol, outputSymbol]) >>> for state in automata.getStates(): ... print(state) State 0 State 1 State 2
>>> for state in automata.getStates(main_states=True): ... print(state) State 1
- getTransition(name)[source]#
Returns the Transition object of the given name.
- Parameters
name (
str
, required) – The name of the Transition object- Returns
The Transition object.
- Return type
- Raise
KeyError
if the name is not found.
- getTransitions()[source]#
Return all the transitions of the automaton.
- Returns
A list containing all the automaton transitions.
- Return type
a
list
ofTransition
- set_cbk_read_symbol_timeout(cbk_method, states=None)[source]#
Function called to handle cases where a timeout appears when waiting for a symbol. In a non initiator context, this symbol would correspond to the input symbol that should trigger a transition. In an initiator context, this symbol would correspond to an output symbol that is expected according to the current transition.
- Parameters
cbk_method (
Callable
, required) – A function used to handle the selection of the next state when no symbol is received after the timeout has expired.states (List[State], optional) – A list of states on which the callback function should apply. If no states are specified, the callback function is applied on all states of the automaton.
- Raise
TypeError
ifcbk_method
is not a callable function
The callback function that can be used in the
cbk_method
parameter has the following prototype:- cbk_method(current_state, current_transition)
- Parameters
current_state (State) – Corresponds to the current state in the automaton. It is expected that the current state cannot be
None
, because when an actor visits an automaton, it is always positioned at a state even if it is executing a transition (in such case, the current state is the initial state of the transition).current_transition (Transition) – Corresponds to the current transition in the automaton. It is expected that the current transition may be
None
, especially in a non initiator context, where no transition has been initiated.
- Returns
The callback function should return the next state. For example, to stay at the same state, the callback function would have to return the
current_state
value.- Return type
- set_cbk_read_unexpected_symbol(cbk_method, states=None)[source]#
Function called to handle cases where a symbol is received but not expected. In a non initiator context, this symbol would not match the input symbol of the available transitions. In an initiator context, this symbol would not match the expected output symbols of the current transition.
The method expects some parameters:
- Parameters
cbk_method (
Callable
, required) – A function used to handle the selection of the next state when a unexpected symbol is received.states (List[State], optional) – A list of states on which the callback function should apply. If no states are specified, the callback function is applied on all states of the automaton.
- Raise
TypeError
ifcbk_method
is not a callable function
The callback function that can be used in the
cbk_method
parameter has the following prototype:- cbk_method(current_state, current_transition, received_symbol, received_message, received_structure)
- Parameters
current_state (State) – Corresponds to the current state in the automaton. It is expected that the current state cannot be
None
, because when an actor visits an automaton, it is always positioned at a state even if it is executing a transition (in such case, the current state is the initial state of the transition).current_transition (Transition) – Corresponds to the current transition in the automaton. It is expected that the current transition may be
None
, especially in a non initiator context, where no transition has been initiated.received_symbol (Symbol) – Corresponds to the received symbol.
received_message (
bytes
) – Corresponds to the received raw message.received_structure (
OrderedDict
where keys areField
and values arebytes
) – Corresponds to the received message structure.
- Returns
The callback function should return the next state. For example, to stay at the same state, the callback function would have to return the
current_state
value.- Return type
- set_cbk_read_unknown_symbol(cbk_method, states=None)[source]#
Function called to handle cases where a message is received but does not correspond to a known symbol. In a non initiator context, this message would not match the input symbol of the available transitions. In an initiator context, this message would not match the expected output symbols of the current transition.
The method expects some parameters:
- Parameters
cbk_method (
Callable
, required) – A callable function used to handle the selection of the next state when an unknown symbol is received.states (List[State], optional) – A list of states on which the callback function should apply. If no states are specified, the callback function is applied on all states of the automaton.
- Raise
TypeError
ifcbk_method
is not a callable function
The callback function that can be used in the
cbk_method
parameter has the following prototype:- cbk_method(current_state, current_transition, received_message)
- Parameters
current_state (State) – Corresponds to the current state in the automaton. It is expected that the current state cannot be
None
, because when an actor visits an automaton, it is always positioned at a state even if it is executing a transition (in such case, the current state is the initial state of the transition).current_transition (Transition) – Corresponds to the current transition in the automaton. It is expected that the current transition may be
None
, especially in a non initiator context, where no transition has been initiated.received_message (
bytes
) – Corresponds to the received raw message.
- Returns
The callback function should return the next state. For example, to stay at the same state, the callback function would have to return the
current_state
value.- Return type